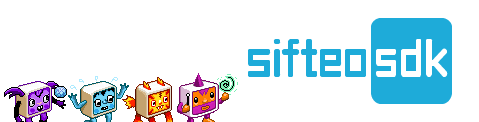 |
v1.1.0
|
28 # error This is a userspace-only header, not allowed by the current build.
31 #include <sifteo/abi.h>
59 #define NOINLINE __attribute__ ((noinline))
76 #define ALWAYS_INLINE __attribute__ ((always_inline))
80 #define STRINGIFY(_x) #_x
90 #define TOSTRING(_x) STRINGIFY(_x)
94 #define SRCLINE __FILE__ ":" TOSTRING(__LINE__)
117 #define DEBUG_ONLY(_x) \
119 if (_SYS_lti_isDebug()) { \
181 if (_SYS_lti_isDebug()) \
182 _SYS_lti_log(__VA_ARGS__); \
205 # define ASSERT(_x) \
207 if (_SYS_lti_isDebug() && !(_x)) { \
208 _SYS_lti_log("ASSERT failure at %s:%d, (%s)\n", __FILE__, __LINE__, #_x); \
227 #define SCRIPT_TYPE(_type) \
229 _SYS_log((_SYS_SCRIPT_ ## _type) | (_SYS_LOGTYPE_SCRIPT << 27), \
266 #define SCRIPT(_type, _code) \
268 if (_SYS_lti_isDebug()) { \
269 SCRIPT_TYPE(_type); \
270 _SYS_lti_log("%s", #_code); \
303 #define SCRIPT_FMT(_type, ...) \
305 if (_SYS_lti_isDebug()) { \
306 SCRIPT_TYPE(_type); \
307 _SYS_lti_log(__VA_ARGS__); \
314 #define LOG_INT(_x) LOG("%s = %d\n", #_x, (_x));
318 #define LOG_HEX(_x) LOG("%s = 0x%08x\n", #_x, (_x));
322 #define LOG_FLOAT(_x) LOG("%s = %f\n", #_x, (_x));
326 #define LOG_STR(_x) LOG("%s = \"%s\"\n", #_x, (const char*)(_x));
330 #define LOG_INT2(_x) LOG("%s = (%d, %d)\n", #_x, (_x).x, (_x).y);
334 #define LOG_INT3(_x) LOG("%s = (%d, %d, %d)\n", #_x, (_x).x, (_x).y, (_x).z);
338 #define LOG_FLOAT2(_x) LOG("%s = (%f, %f)\n", #_x, (_x).x, (_x).y);
342 #define STATIC_ASSERT(_x) ((void)sizeof(char[1 - 2*!(_x)]))
345 #define MIN(a,b) ((a) < (b) ? (a) : (b))
348 #define MAX(a,b) ((a) > (b) ? (a) : (b))
360 #define arraysize(a) (sizeof(a) / sizeof((a)[0]))
366 #define offsetof(t,m) ((uintptr_t)(uint8_t*)&(((t*)0)->m))